Grails + AngularJS + CoffeeScript
Intro
Responsive design is a must for modern web-applications. Today this is attempted to achieve by using JavaScript MVC frameworks.
But more often than not those frameworks originate from Rails
, NodeJS
and other non-Java communities.
This makes it hard for Java-boys (as me) to jump into. So purpose of this short tutorial is to explain in few easy steps how to start with JS MVC framework and CoffeeScript
on Grails
.
In this certain case AngularJS
is my personal choice taken up after playing around with few other libraries but principles described below should work for other frameworks.
Our intention will be to write one-page application having single input field and what’s typed there is immediately reflected below in span tag.
I’m assuming readers could be unfamiliar with AngularJS, but, still, the application is really simple and should be easy to read.
Implementation
- First let’s create Grails application
-
grails create-app testApp
- After application is created let’s change folder and all subsequent commands should be executed there
-
cd testApp
Grails
already has plugin that support on-the fly conversion of yourCoffeeScript
files intoJavaScript
. To install it type following-
grails install-plugin coffeescript-resources
- After plugin installed we are going to create empty file for our
CoffeeScript
sources -
web-app/cs/angularIndex.coffee
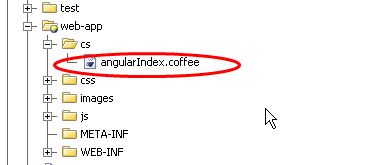
- What’s great about Grails - is the fact that it abounds with plugins.
AngularJS
library can be installed by typing -
grails install-plugin angularjs-resources
- Thanks to great
resources
pluginJavaScript
files management is quite easy inGrails
modules = {
angularIndex {
dependsOn 'angular'
resource url: 'cs/angularIndex.coffee'
}
}
Code above declares resource for our AngularJS module written in CoffeeScript
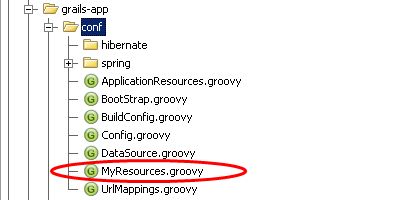
- Next we need controller to serve starting point HTML with AngularJS module. We can do it with following command
-
grails create-controller AngularIndex
Also we need manually create Grails view for index action of our controller. For this we have to create new file with following content
.grails-app/views/angularIndex/index.gsp
<!DOCTYPE html>
<html>
<head>
<r:require modules="angularIndex"/>
<r:layoutResources/>
</head>
<body ng-app="MyApp">
<div ng-controller="MyCtrl">
What typed reflected below
<input type="text" ng-model="myText"/>
<span><b>{{myText}}</b></span>
</div>
</body>
<r:layoutResources/>
</html
- Last step is to create AngularJS module in CoffeeScript. For this we have to fill
web-app/cs/angularIndex.coffee
created before with following content
app = angular.module "MyApp", []
app.controller "MyCtrl", ($scope) ->
$scope.myText = "INITIAL"
- Let’s run application
-
grails run-app
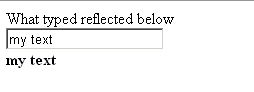